Vite.js 读取环境变量误区 作者: Shine 时间: 2022-09-17 分类: TypeScript,WEB开发 评论 ## 背景 当我在打包文件后,调试时发现我的环境变量都输出在 JS 中,当时我大为震惊? 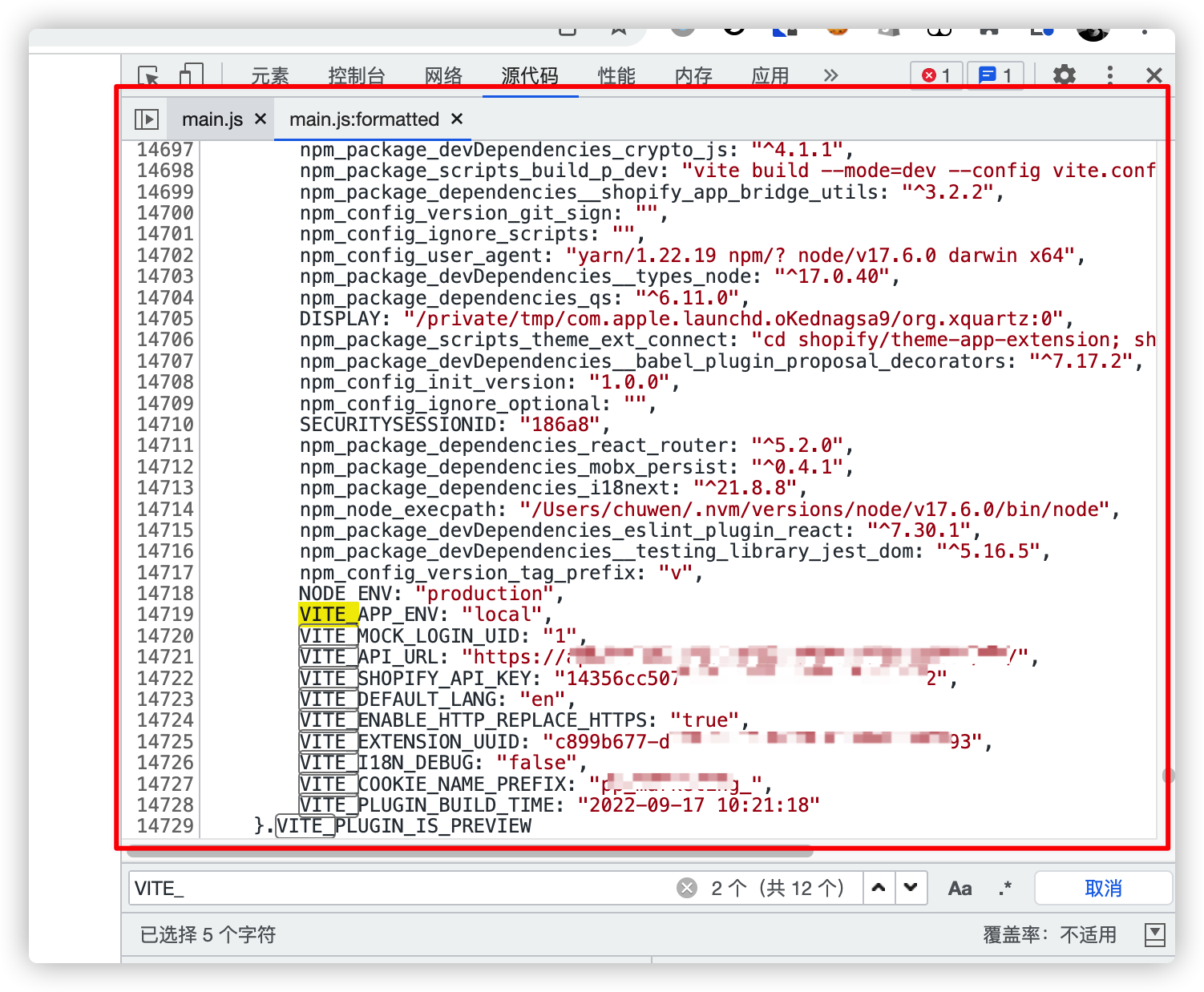 ## 解决办法 然后仔细回想,我用的是 `process.env` 去读取环境变量的,我记得在 Vite.js 官方文档中说,只会暴露出 `VITE_` 前缀的环境变量,带着这个疑惑,我又翻看了官方文档:https://cn.vitejs.dev/guide/env-and-mode.html 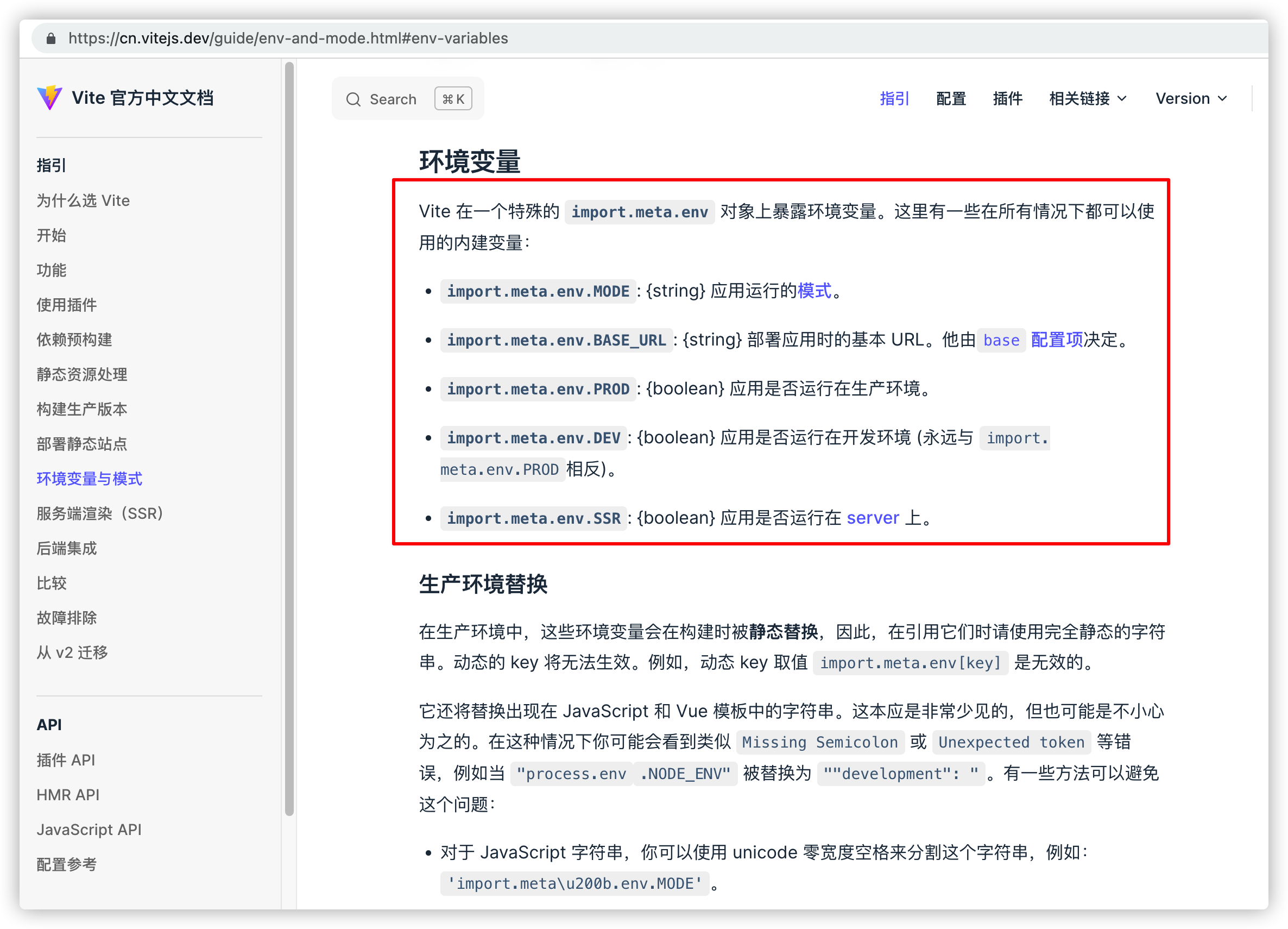 ### 恍然大悟 原来是我记错了/搞混了,应该是用 `import.meta.env` 去读取环境变量,然后立即全局替换,将`process.env.` 替换为 `import.meta.env` ### 再次打包 再次打包预览后,发现只剩下 `VITE_` 前缀的环境变量了 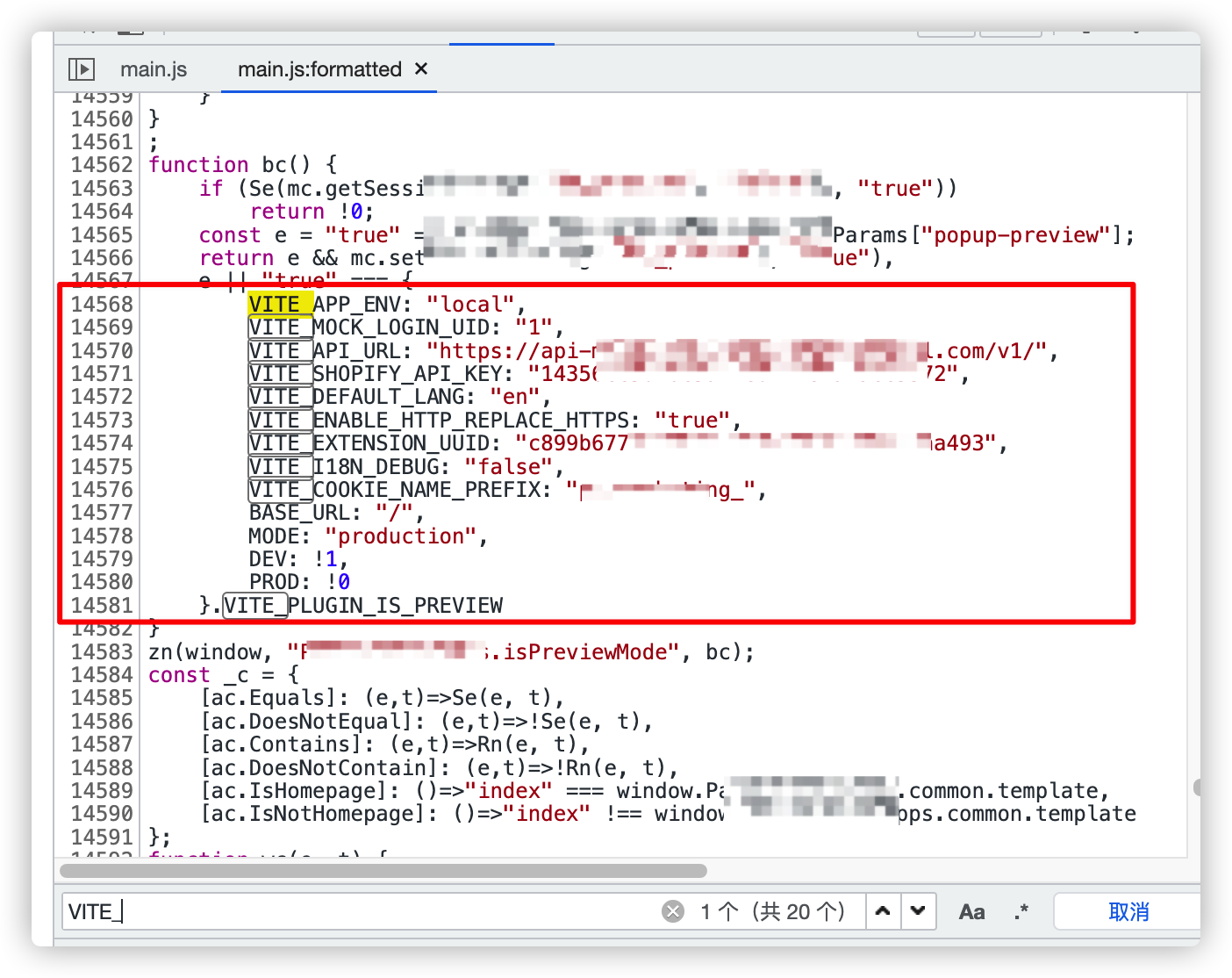 ## 可优化的点 我发现打包后,只要在任意地方用到了 `import.meta.env.VITE_*`,就会打包成这样,所以我在很多地方都能够看到 ```js var isPreview = "true" === { VITE_APP_ENV: "local", VITE_MOCK_LOGIN_UID: "1", VITE_API_URL: "https://api-.com/v1/", VITE_SHOPIFY_API_KEY: "14356af9cc5372", VITE_DEFAULT_LANG: "en", VITE_ENABLE_HTTP_REPLACE_HTTPS: "true", VITE_EXTENSION_UUID: "c899b-afa8917aa493", VITE_I18N_DEBUG: "false", VITE_COOKIE_NAME_PREFIX: "pg_", BASE_URL: "/", MODE: "production", DEV: !1, PROD: !0 }.VITE_PLUGIN_IS_PREVIEW ``` 这显然不是很好的做法 ### 优化办法 对此我想到一个简单的优化办法,可以写成如这种: ```ts /* eslint-disable sort-keys,sort-keys-fix/sort-keys-fix */ const envLists = import.meta.env const EnvHelper = { // 自己定义的 env: envLists.VITE_APP_ENV, // vite 自带的判断条件 get mode() { return envLists.MODE }, get isDev() { return envLists.MODE === "development" }, get isProd() { return !this.isDev }, // ///////// // 自定义 get mockLoginUid() { return envLists.VITE_MOCK_LOGIN_UID }, get apiURL() { return envLists.VITE_API_URL }, get shopifyApiKey() { return envLists.VITE_SHOPIFY_API_KEY }, get defaultLang() { return envLists.VITE_DEFAULT_LANG }, get enableHttpReplaceHttps() { return envLists.VITE_ENABLE_HTTP_REPLACE_HTTPS }, get extensionUUID() { return envLists.VITE_EXTENSION_UUID }, get i18nDebug() { return envLists.VITE_I18N_DEBUG }, get cookieNamePrefix() { return envLists.VITE_COOKIE_NAME_PREFIX }, get pluginIsPreview() { return envLists.VITE_PLUGIN_IS_PREVIEW }, get pluginBuildTime() { return envLists.VITE_PLUGIN_BUILD_TIME } } export default EnvHelper ```
解决 Laravel 使用 asset 函数加载资源为 http 协议 / Laravel Horizon 加载资源为 http 协议 作者: Shine 时间: 2022-09-14 分类: Laravel 评论 ## 背景 搭建了个 horizon,使用 https 打开,但是打开的界面是下图所示 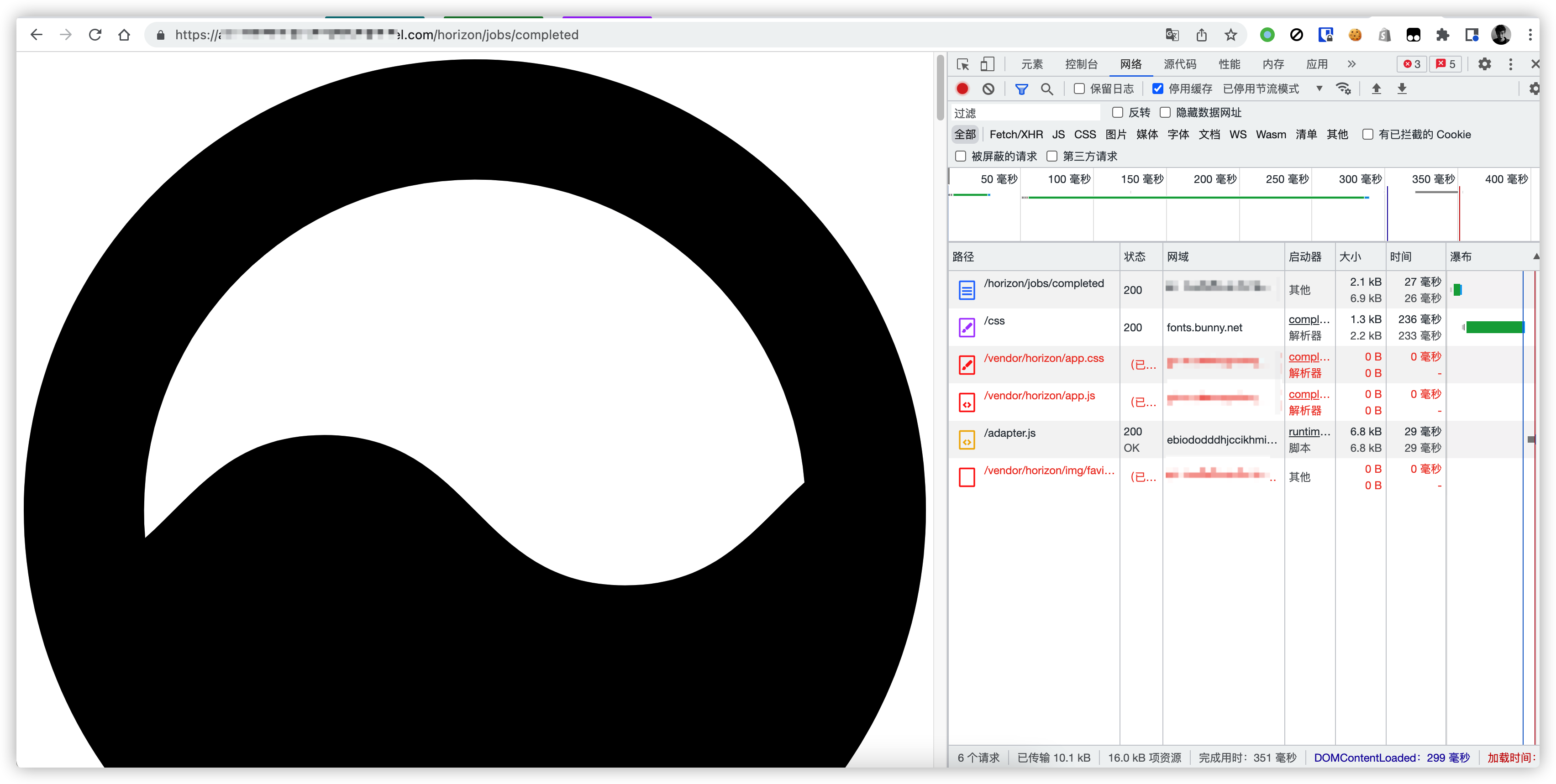 一看加载的资源是 http 的,那难怪页面无法正常加载 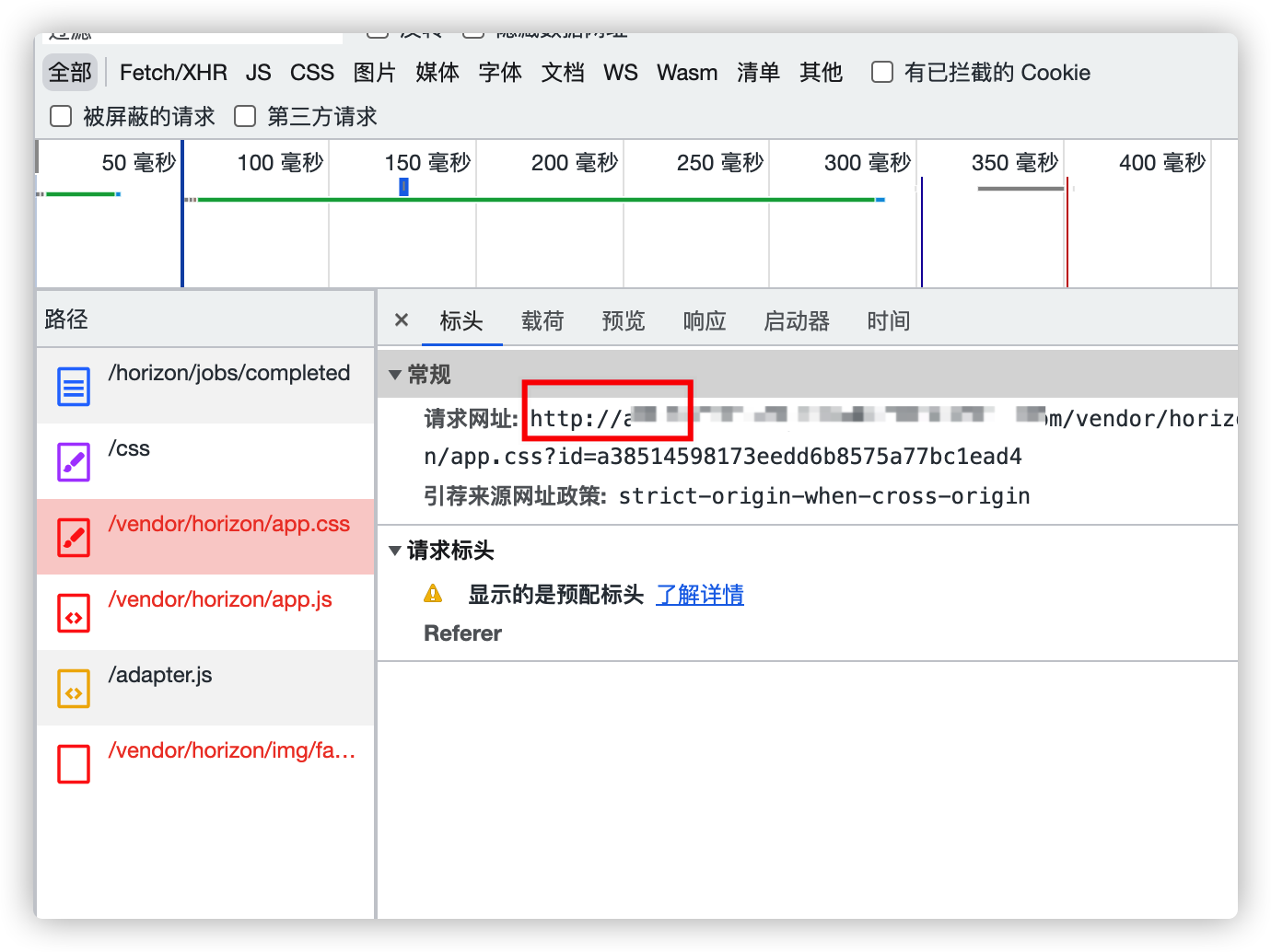 ## 解决办法 在 Google 搜索中,找到了这条答案:https://stackoverflow.com/a/68287406 我认为这才是最标准的解决方案 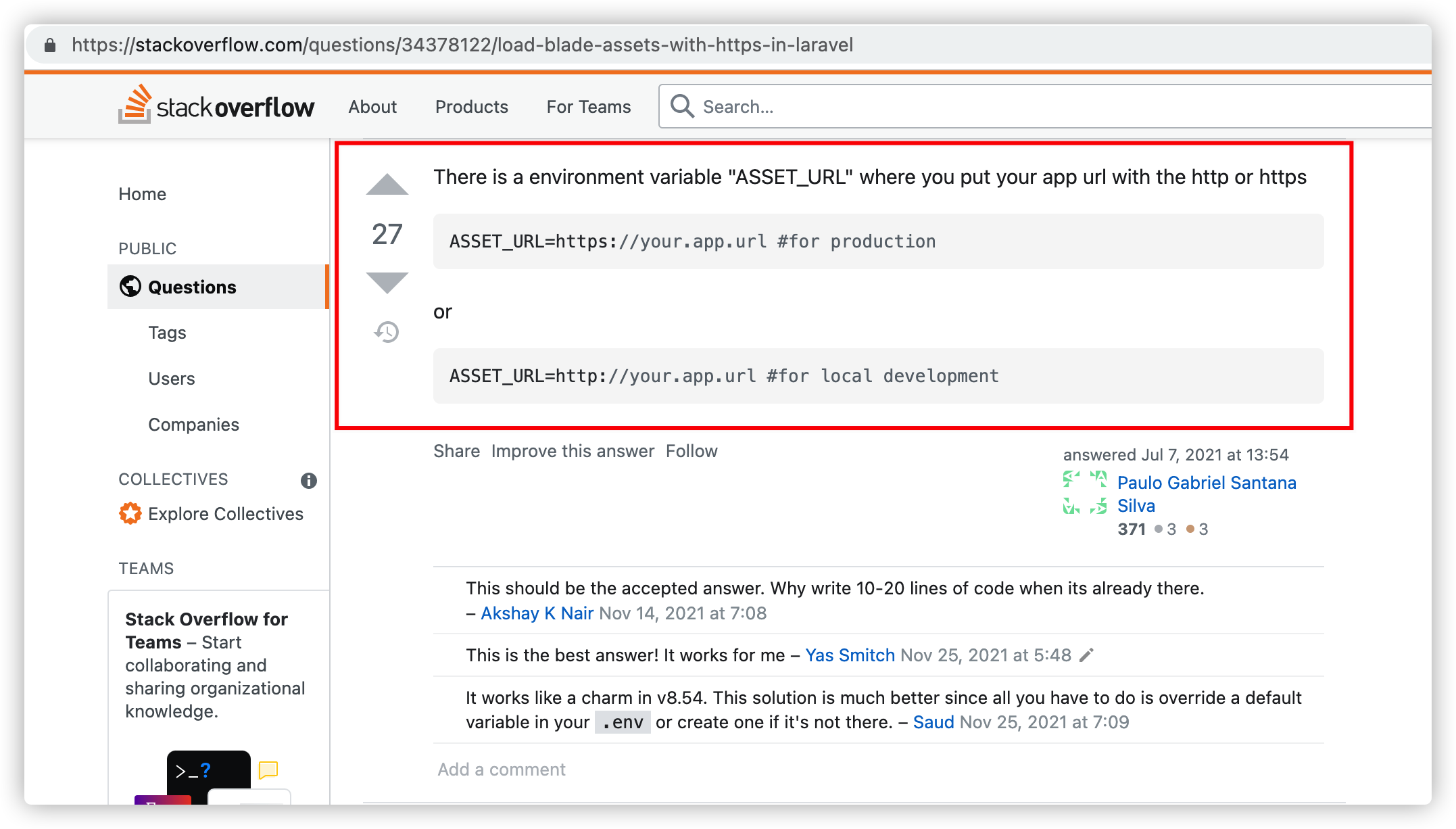 比起那些去改 `AppServiceProvider.php` 靠谱多了 ### 总结下用法 你只需要在 `.env` 文件里配置号 `ASSET_URL` 环境变量即可,像我这样 ```env ASSET_URL=https://cdn.nowtime.cc ``` 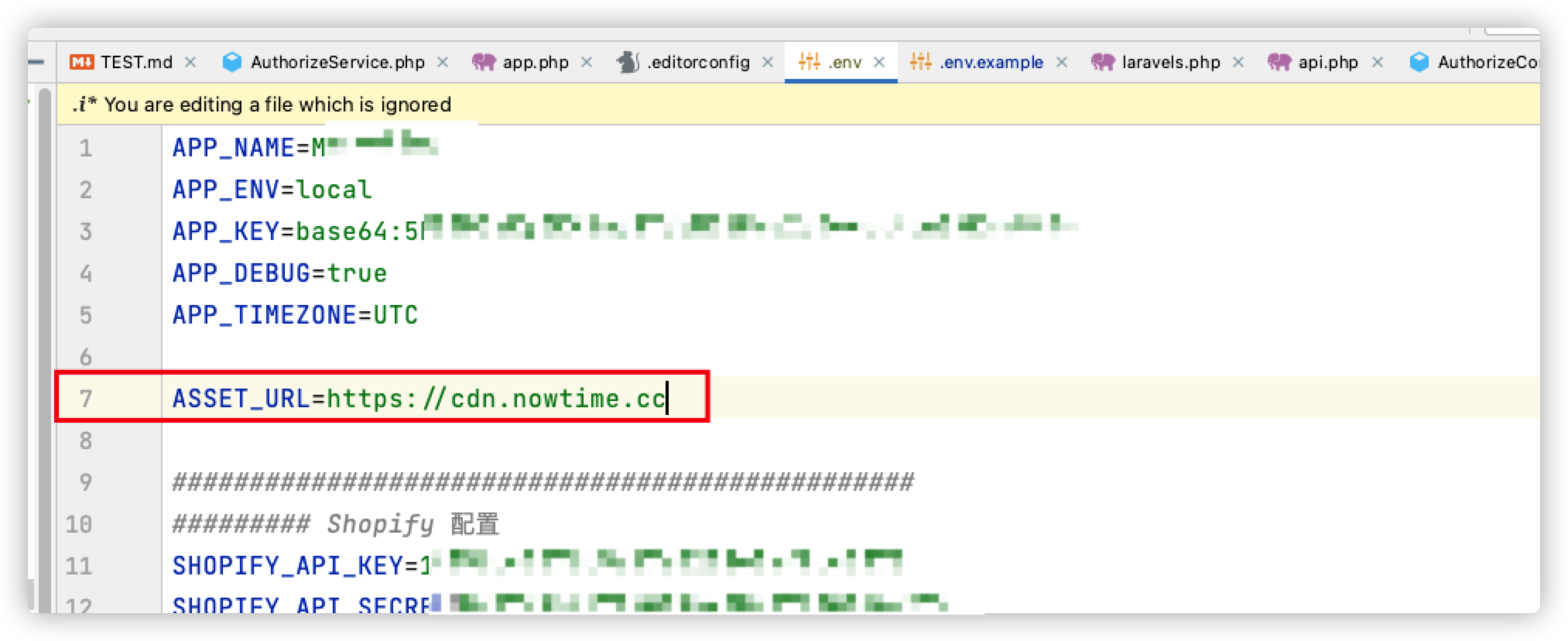 ### 完美解决 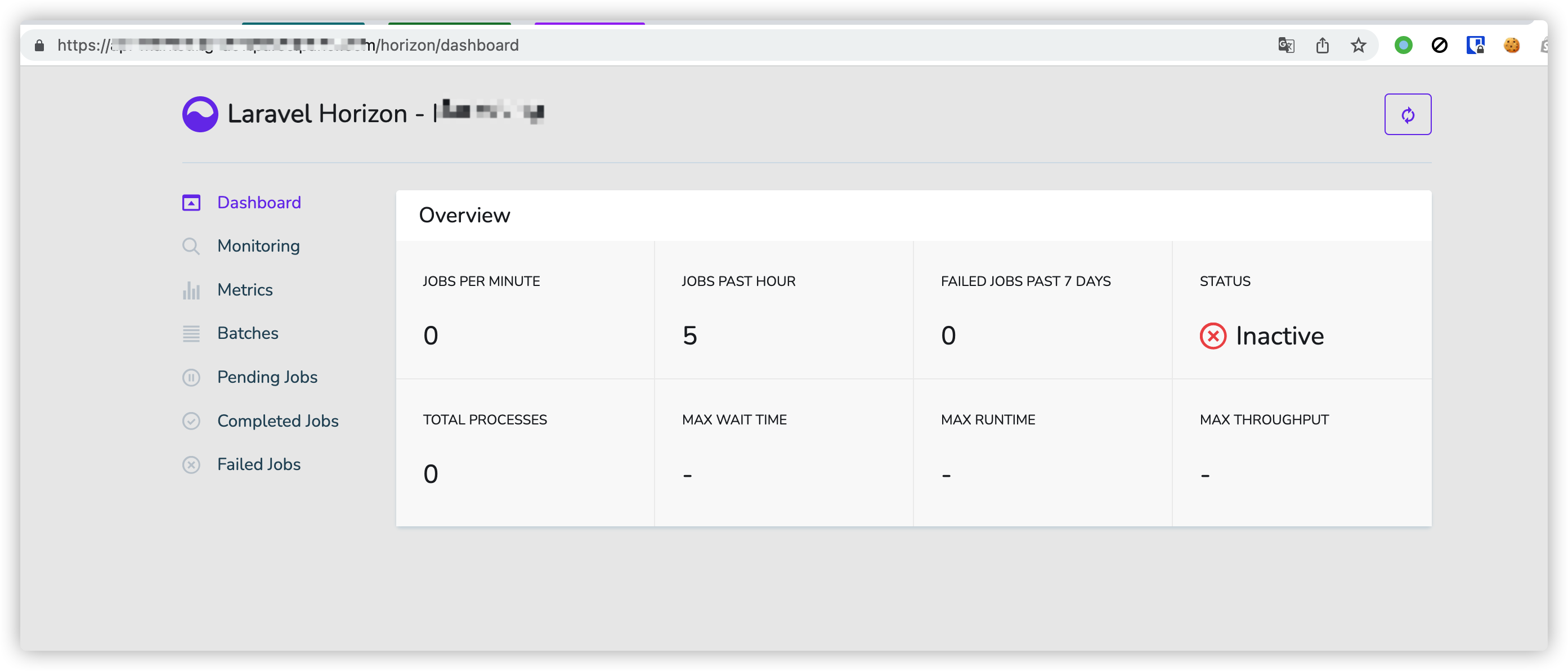
解决 GitHub Connect: kex_exchange_identification: Connection closed by remote host 作者: Shine 时间: 2022-07-30 分类: Linux 评论 # 背景 想要克隆项目/推送代码到 GitHub 老实错误,检查发现: ```shell ➜ ~ ssh -vvv git@github.com OpenSSH_8.1p1, LibreSSL 2.7.3 debug1: Reading configuration data /Users/chuwen/.ssh/config debug1: /Users/chuwen/.ssh/config line 1: Applying options for * debug1: /Users/chuwen/.ssh/config line 6: Applying options for github.com debug1: Reading configuration data /etc/ssh/ssh_config debug1: /etc/ssh/ssh_config line 47: Applying options for * debug1: Executing proxy command: exec nc -v -x 127.0.0.1:7890 github.com 443 debug1: identity file /Users/chuwen/.ssh/id_rsa type 0 debug1: identity file /Users/chuwen/.ssh/id_rsa-cert type -1 debug1: identity file /Users/chuwen/.ssh/id_dsa type -1 debug1: identity file /Users/chuwen/.ssh/id_dsa-cert type -1 debug1: identity file /Users/chuwen/.ssh/id_ecdsa type -1 debug1: identity file /Users/chuwen/.ssh/id_ecdsa-cert type -1 debug1: identity file /Users/chuwen/.ssh/id_ed25519 type -1 debug1: identity file /Users/chuwen/.ssh/id_ed25519-cert type -1 debug1: identity file /Users/chuwen/.ssh/id_xmss type -1 debug1: identity file /Users/chuwen/.ssh/id_xmss-cert type -1 debug1: Local version string SSH-2.0-OpenSSH_8.1 Connection to github.com port 443 [tcp/https] succeeded! kex_exchange_identification: Connection closed by remote host ``` # 解决方案 最后找到[一篇文章](https://idreamshen.github.io/posts/github-connection-closed/ "一篇文章")解决了这个问题。 只需要在 `~/.ssh/config` 配置以下项目即可 ```config Host github.com HostName ssh.github.com User git Port 443 ``` 如果想要配置代理可以这样: ```config Host github.com HostName ssh.github.com User git Port 443 # 走 HTTP 代理 # ProxyCommand socat - PROXY:127.0.0.1:%h:%p,proxyport=8080 # 走 socks5 代理(如 Shadowsocks) ProxyCommand nc -v -x 127.0.0.1:7890 %h %p ``` ## 最终解决了问题 ```shell ➜ ~ ssh -T git@github.com Connection to ssh.github.com port 443 [tcp/https] succeeded! Hi PrintNow/react-i18next-base-usage! You've successfully authenticated, but GitHub does not provide shell access ```
Laravel Middleware(中间件) 设置登录信息(User Model) 作者: Shine 时间: 2022-06-29 分类: Laravel,PHP 评论 Laravel Middleware(中间件) 设置登录信息(User Model),然后在其它地方使用如 Controller(控制器) ### UserAdminAuthGuard 中间件 ```php firstOrFail()); return $next($request); } } ``` ### UserController - HTTP 控制器 ```php
Laravel 控制器测试 作者: Shine 时间: 2022-06-02 分类: Laravel,PHP 评论 ## 新建控制器名字为 ForExampleController.php ```php get('domain')) { return response([ 'code' => 400, 'msg' => '错误的参数', ]); } return response([ 'code' => 200, 'msg' => '验证通过', ]); } } ``` ## 新建测试文件名字为 ForExampleControllerTest.php ```php 'nowtime.cc', ]); $forExampleController = new ForExampleController(); $res = json_decode($forExampleController->index($request)->getContent(), true); self::assertEquals(200, $res['code']); } } ``` 运行测试结果: 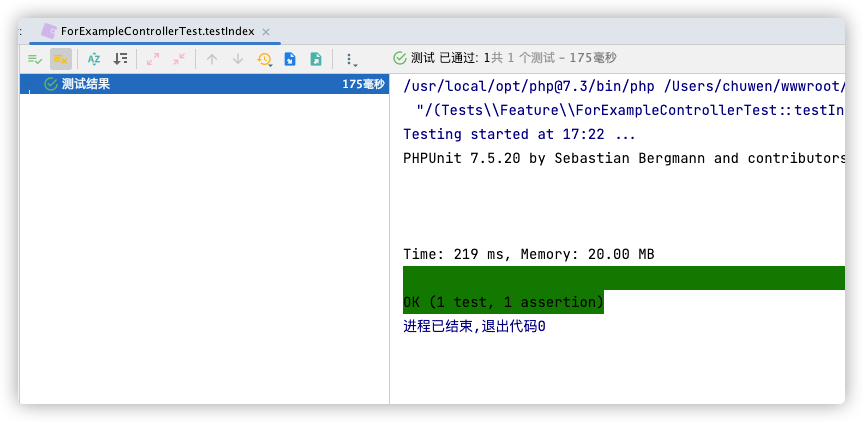