Laravel `Http::withUrlParameters` 用法 作者: Chuwen 时间: 2023-07-18 分类: Laravel,PHP 评论 ## 使用前 在调用 HTTP API 时会发现有很多 API 都是采用 *REST API* 风格,例如: * /admin/api/2023-07/products/{product_id}.json * /admin/api/2023-01/orders/{order_id}.json * /admin/api/2023-01/orders/{order_id}/fulfillments/{fulfillment_id}.json * ... 不难发现它们都有一个共同点,以花括号(`{var_name}`、`{order_id}`)包裹的变量替换为对应的值,通常我们可能会这么做: ```php $http = \Illuminate\Support\Facades\Http::baseUrl('https://example.com'); $order_id = 123456789; $fulfillment_id = 9889894445; $orderUrl = sprintf('/admin/api/2023-07/orders/%d.json', $product_id); $fulfillmentUrl = sprintf('/admin/api/2023-01/orders/%d/fulfillments/%d.json', $order_id, $fulfillment_id); $order = $http->get($orderUrl); $fulfillment = $http->get($fulfillmentUrl); ``` 但是会发现这样拼接 URI 会很繁琐 ## 使用后 - `Http::withUrlParameters` ```php $http = \Illuminate\Support\Facades\Http::baseUrl('https://example.com') ->withUrlParameters([ 'order_id' => 123456789, 'fulfillment_id' => 9889894445 ]); // 比如你要使用上述定义 URL 参数:order_id // 你在 URL 只需要填 {order_id} // 在请求时会自动替换 $orderUrl = '/admin/api/2023-07/orders/{order_id}.json'; $fulfillmentUrl = '/admin/api/2023-01/orders/{orders_id}/fulfillments/{fulfillment_id}.json'; $order = $http->get($orderUrl); $fulfillment = $http->get($fulfillmentUrl); ``` 会发现使用会很方便,不需要再使用如 `sprintf` 去拼接 URL 了
Laravel 模型(Model) 关联(eloquent-relationships) 查询未找到值返回默认值 作者: Chuwen 时间: 2023-05-29 分类: Laravel 评论 ## Laravel 模型关联查询未找到值返回默认值 在实际业务中,经常会用到模型关联查询,但是有时候关联的那个模型在数据库中不存在,则会返回 null,如果你再去判断是否为 null 逻辑就很复杂,下面给出一个现实中的常见一个例子 假设有 2 个模型,`User::class` 和 `UserConfig::class` **User::class** ```php hasOne(\App\Models\UserConfig::class, 'uid', 'id'); } } ``` **UserConfig::class** ```php $userM->config->locales ]; ``` ## 解决方法 [翻阅文档](https://laravel.com/docs/10.x/eloquent-relationships#default-models)发现有这样一个方法 `withDefault()` 官方文档的解释: > The belongsTo, hasOne, hasOneThrough, and morphOne relationships allow you to define a default model that will be returned if the given relationship is null. This pattern is often referred to as the Null Object pattern and can help remove conditional checks in your code. In the following example, the user relation will return an empty App\Models\User model if no user is attached to the Post model: > > 机器翻译成中文: > >> 如果给定的关系为空,则可以定义默认模型。这种模式通常称为 `空对象模式`,可以帮助删除代码中的条件检查。在以下示例中,如果没有用户附加到Post模型,用户关系将返回一个空的App\Models\User模型: 用法很简单,只需要在 `hasOne` 末尾加上 `->withDefault()` 方法,然后在 `UserConfig::class` 的 `$attributes` 属性写上默认值即可 **User::class** ```php hasOne(\App\Models\UserConfig::class, 'uid', 'id')->withDefault(); } } ``` **UserConfig::class** ```php ['en'] ]; } ``` ```php $uid = 1; $userM = User::findOrFail($uid); return [ // 如果关联的 config 模型查找不到值,就会返回默认值 ['en'] 'locales' => $userM->config->locales ]; ```
Laravel Model(模型)实现分表查询 - 根据 UID 一定数量分表查询 作者: Chuwen 时间: 2023-04-28 分类: Laravel,PHP 评论 当业务达到一定量,一些表就可能需要用到分表、分库等方法,加快数据库查询速度,在 Laravel 中可以按照如下方式实现 ### 代码实现如下 ```php table . "_" . (($tableNum - 1) * $count + 1) . "_" . ($tableNum * $count); } public function scopeByUid($query, int $uid) { return $query->from(self::getPartitionedTableName($uid))->where('uid', $uid); } } ``` ### 使用方法 ```php // 查找的表是 orders_1_10000 Orders::byUid(4)->first(); // 查找的表是 orders_10001_20000 Orders::byUid(20000)->first(); ```
使用 PHPStorm 的高级元数据(PhpStorm advanced metadata) 作者: Chuwen 时间: 2023-02-11 分类: Laravel,PHP 评论 ## 例子 1 你通过 `$request->user()` 想拿到用户模型,但是没有类型提示很苦恼,你可以这样做 在项目跟路径创建一个文件 `.phpstorm.meta.php`,然后里面的内容写: ```php \App\Models\User::class, ])); } ``` 然后就会有类型提示了: 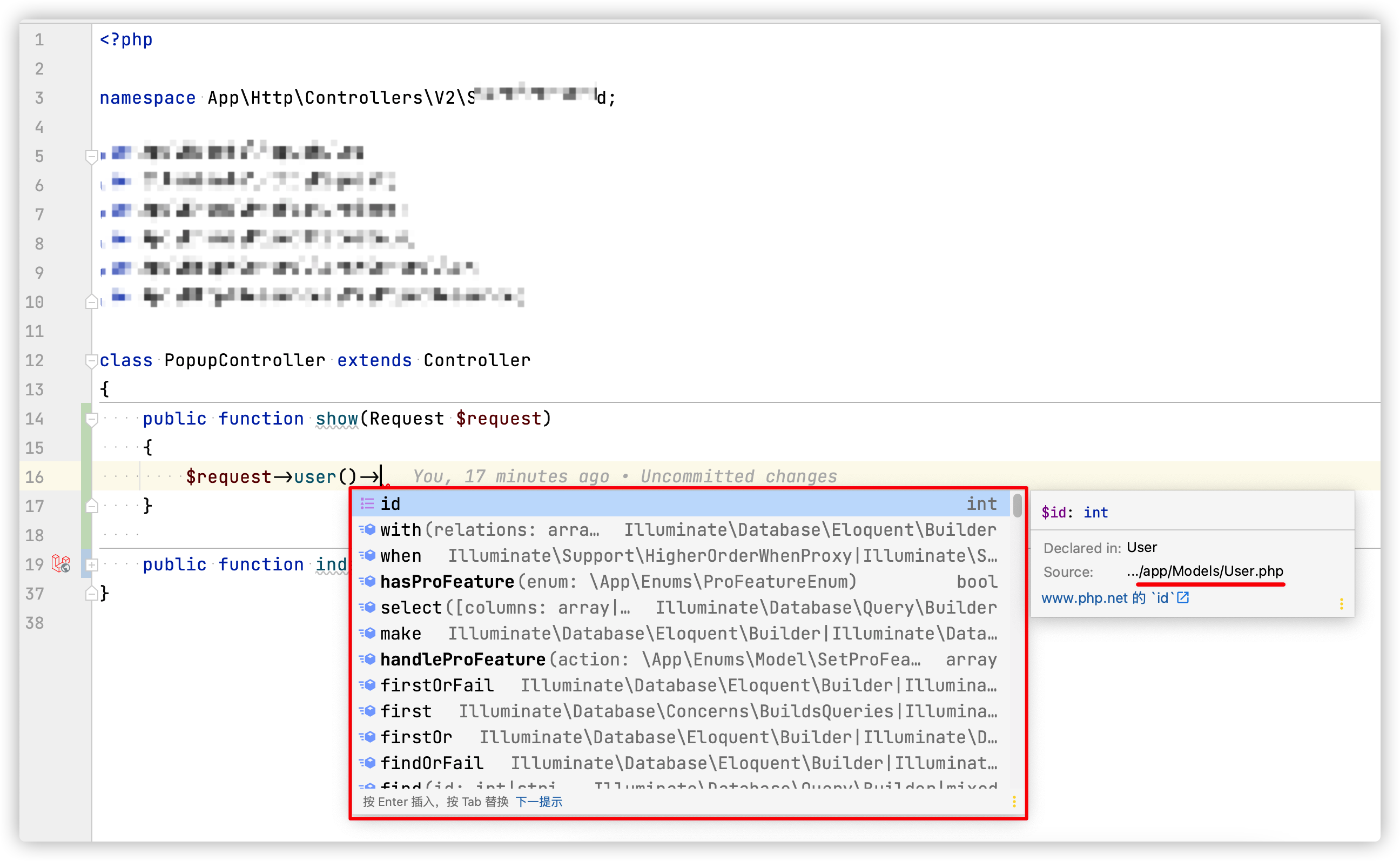 更多高级用法请看:https://www.jetbrains.com/help/phpstorm/ide-advanced-metadata.html#map
Laravel 根据 Session ID 获取相关值 作者: Chuwen 时间: 2022-10-10 分类: Laravel 评论 ## 背景 - 「A 系统」域名 `admin.xxxx.com` - 使用的是 Dcat Admin,Session 身份认证 - 「A1 系统」域名 `api.sass.com` - 使用 JWT 身份认证 因为项目有个需求,需要从「A 系统」访问「A1 系统」,实际上是「A 系统」生成 JWT 传递给「A1 系统」,JWT 格式如下: > 使用 `sub` 标记是谁生成的,让「A1 系统」能够进行判断 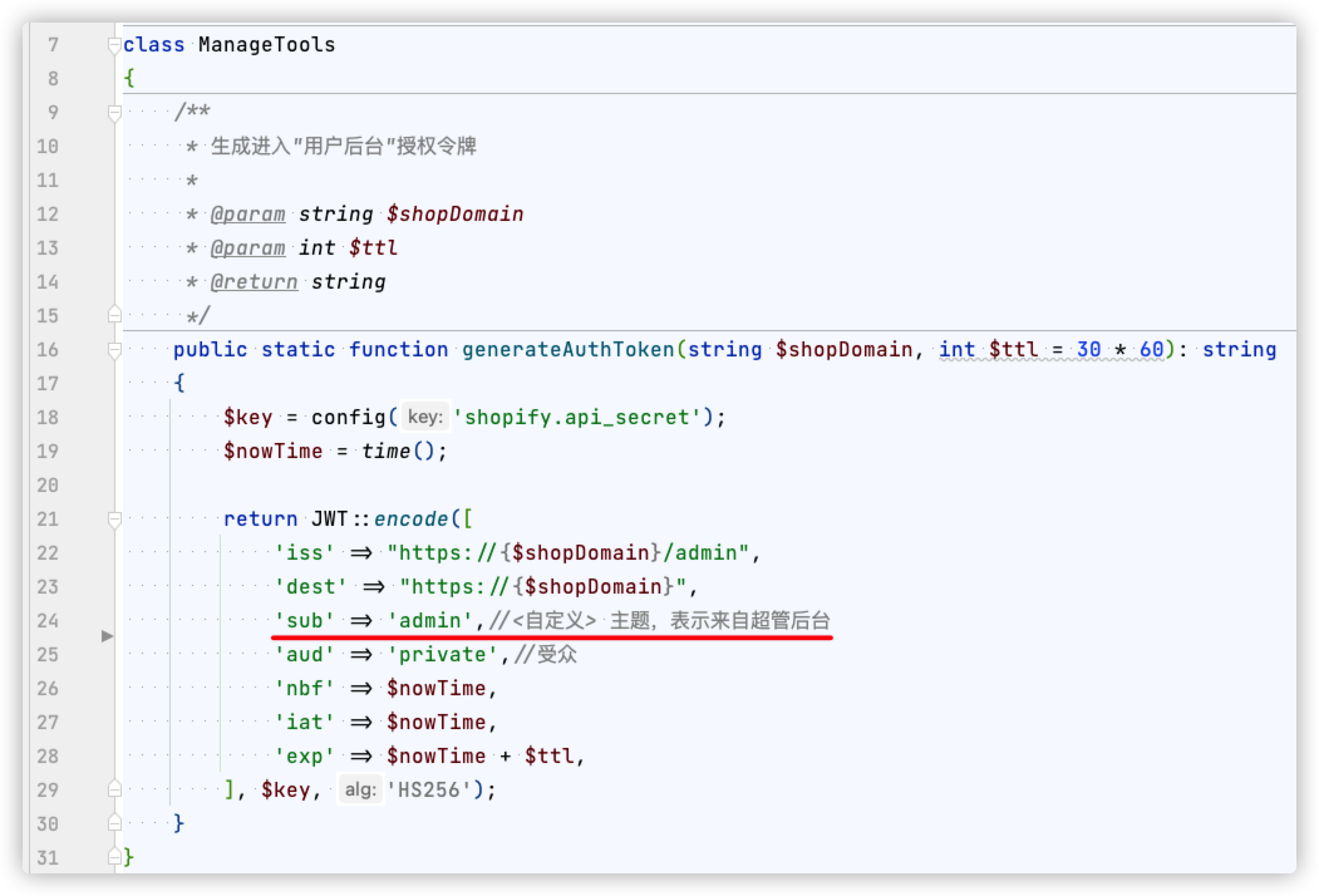 需要实现「A 系统」退出登录,「A1 系统」的 JWT 信息就不能通过认证 因为这 2 个系统是共用一套代码的,所以想到了使用 `Session ID` 写入 JWT `payload` 中,然后「A1 系统」拿到 `Session ID` 去校验 ## 实现 ### 拿到 Laravel Session ID 「A 系统」通过 `Illuminate\Support\Facades\Session::getId()` 拿到长度为 40 的 `Session ID`,生成 JWT 传递给「A1 系统」使用 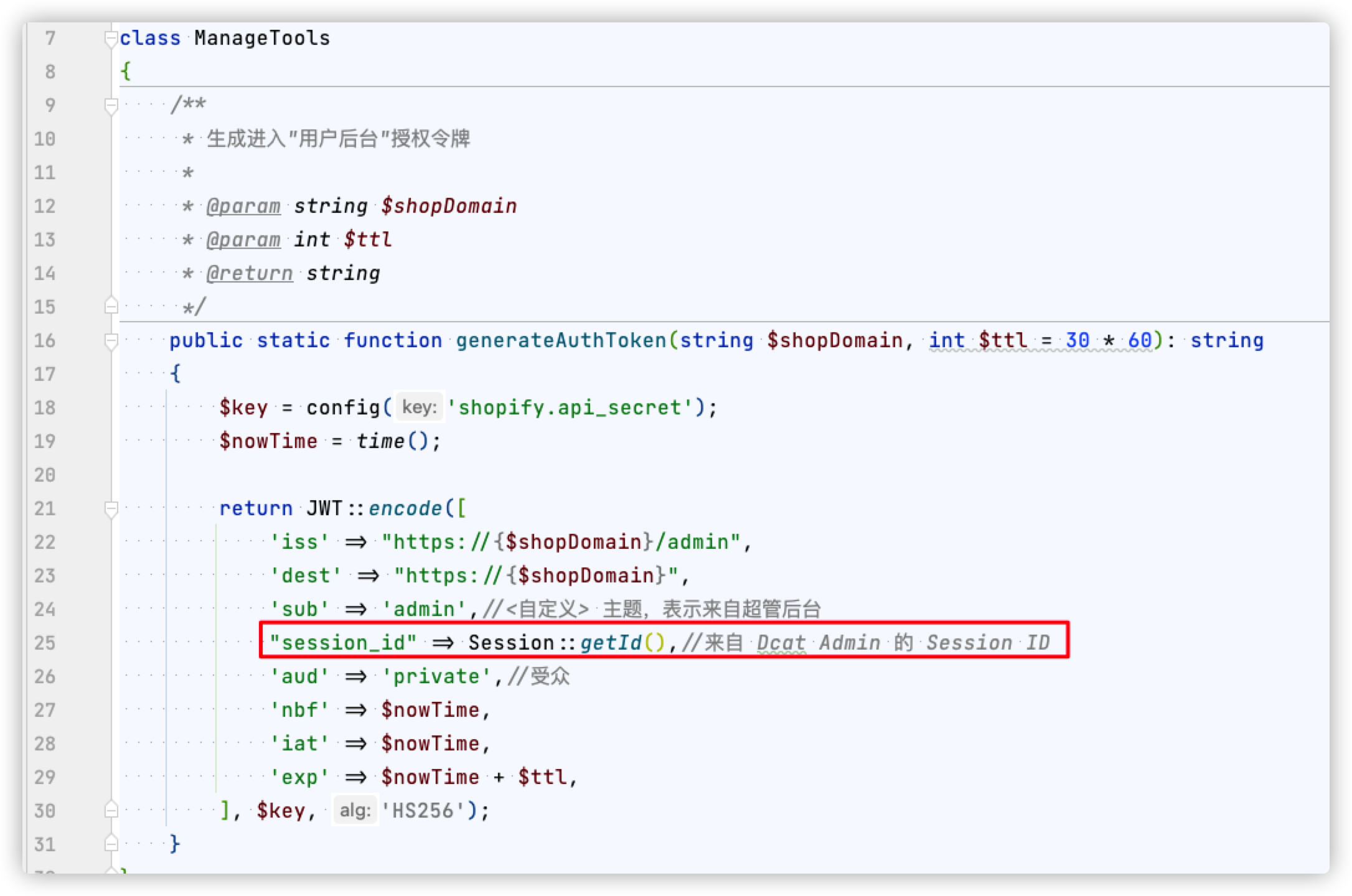 ### 「A1 系统」校验 Session ID 是否存在 示例代码如下: ```php // 这里实际需要替换为从 JWT 解析,拿到 `session_id` 字段 $sessionId = ''; $data = Illuminate\Support\Facades\Session::getHandler()->read($sessionId); if(empty($data)){ // TODO: SessionID 不存在,请检查 } ```