记录一次 Node.js 构建失败的坑 (/bin/sh: vite:未找到命令 error Command failed with exit code 127.) 作者: Shine 时间: 2023-10-13 分类: WEB开发 评论 ## 问题排查 经过排查是 `vite` 包没安装上,然后我就想到了 `vite` 包是写在 `package.json` 文件的 `devDependencies` 清单中 最终查看 [yarn 文档](https://classic.yarnpkg.com/lang/en/docs/cli/install/#toc-yarn-install-production-true-false)发现一个配置项: 如果环境变量 `NODE_ENV` 设置为 `production`,则会忽略 `devDependencies` 清单中的包 然后看了一眼流水线环境变量配置,果不其然设置了 `NODE_ENV=production` 这个环境变量,这也印证了为什么构建时提示 `vite` 命令找不到 ## 解决方案 以下任选一个方案即可 ### 1. 将 NODE_ENV 环境变量删除 ### 2. 安装依赖时追加 `--production=false` 参数 完整示例:`yarn install --production=false`
Tailscale 搭建 derper, 使用 Nginx 反向代理遇到的坑: http: TLS handshake error from 172.40.0.1:44442: cert mismatch with hostname: "" 作者: Shine 时间: 2023-10-10 分类: 谈天说地 评论 ## 序言 按照网上找到的教程,大部分都是让你这么配置 Nginx: ```nginx location / { proxy_pass https://127.0.0.1:3443; proxy_http_version 1.1; proxy_set_header Host $host; proxy_set_header Upgrade $http_upgrade; proxy_set_header Connection "upgrade"; proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for; } ``` 但是这样做你会发现根本访问不了,derper 也会报出如下错误:  cert mismatch with hostname(证书与主机名不匹配) **异常原因(这是我的个人猜测,欢迎 dalao 们指正):** 可能是在 Nginx 在与后端 TLS 握手期间,deper 进行了对 SSL 强校验,发现 hostname 和证书的主机名不匹配,则拒绝连接 ## 解决方案 ```nginx location / { proxy_pass https://127.0.0.1:3443; proxy_http_version 1.1; proxy_set_header Host $host; # 加上这 2 行配置即可解决 proxy_ssl_server_name on; proxy_ssl_name $host; proxy_set_header Upgrade $http_upgrade; proxy_set_header Connection "upgrade"; proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for; } ``` 如果访问你的 derper 地址,出现以下界面表示配置成功 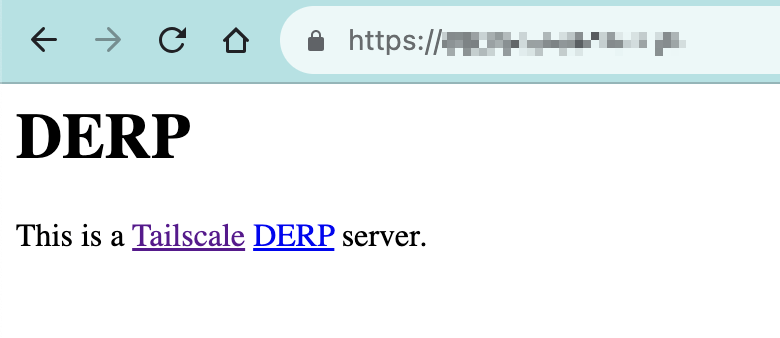
Tailscale 打洞失败解决方案 作者: Shine 时间: 2023-10-09 分类: 唠嗑闲聊 评论 添加一行这个配置 `"randomizeClientPort": true` 就可以了 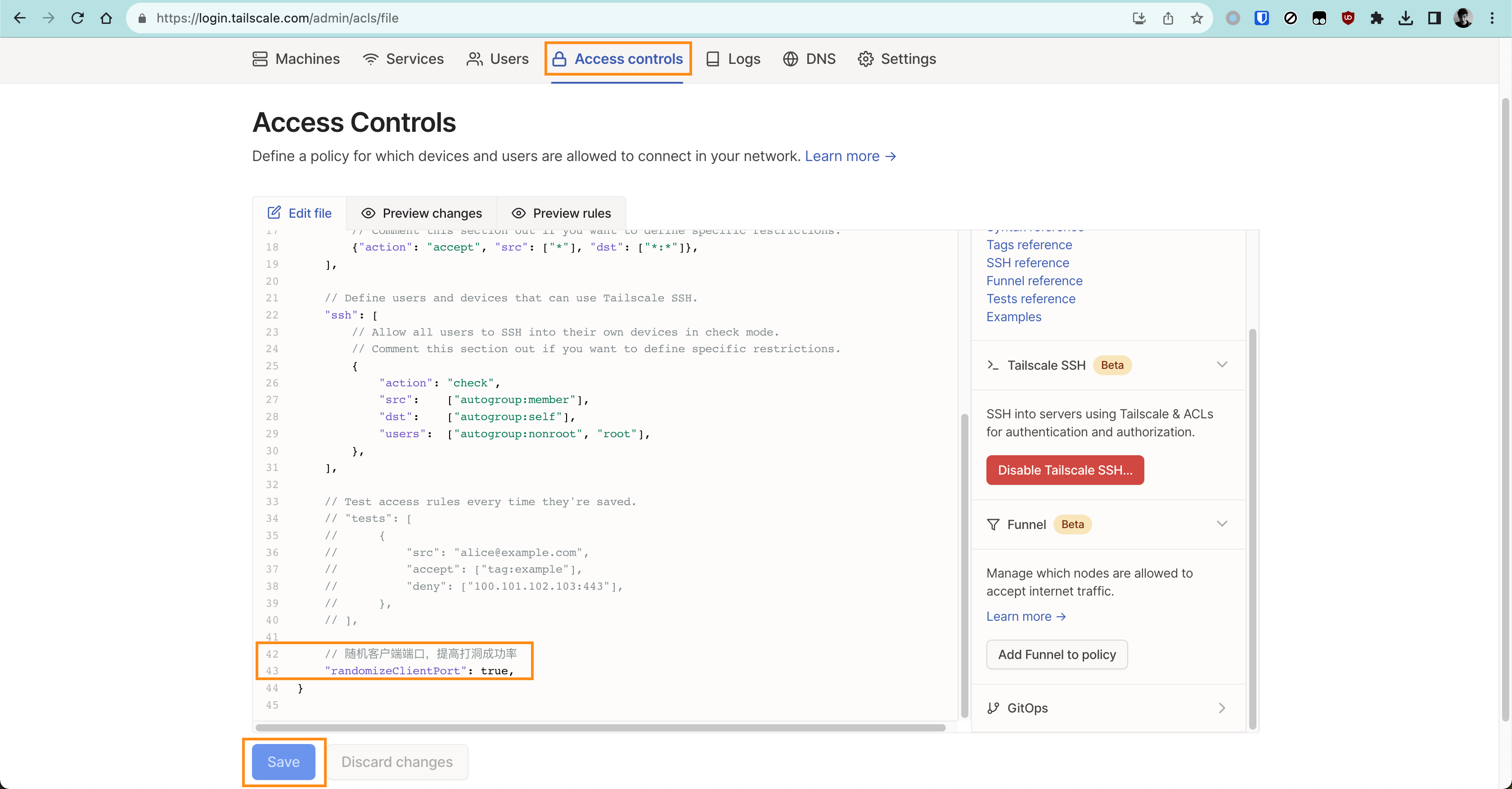
PHP 8.1 Enum (枚举) 根据名字取得枚举 作者: Shine 时间: 2023-09-21 分类: PHP 评论 灵感来源于:https://github.com/php/php-src/issues/9352#issuecomment-1216839118 ```php $enumClass * @param string $name * @return T|null */ function tryFromName(string $enumClass, string $name) { $constName = $enumClass . '::' . $name; return is_subclass_of($enumClass, UnitEnum::class) && defined($constName) ? constant($constName) : null; } // enum(Gender::UNKNOWN) var_dump(tryFromName(Gender::class, 'UNKNOWN')); // enum(Gender::MALE) var_dump(tryFromName(Gender::class, 'MALE')); // enum(Gender::FEMALE) var_dump(tryFromName(Gender::class, 'FEMALE')); // NULL var_dump(tryFromName(Gender::class, 'null')); ```
php-cs-fix 只格式化已经修改的代码 / 只格式化某次提交的代码 作者: Shine 时间: 2023-09-21 分类: PHP 评论 ## 前提 注意需要在 `composer.json` 中的 `scripts:` 部分添加 `"cs-fix": "php-cs-fixer fix $1"`,如下示例 ```json { "name": "hyperf/hyperf-skeleton", "type": "project", // ... "autoload": { "psr-4": { "App\\": "app/" }, "files": [] }, "scripts": { // ...... "cs-fix": "php-cs-fixer fix $1", // ...... }, } ``` ## 命令 ### 只格式化已经修改的代码 ```shell git diff --name-only --cached | grep '\.php$' | xargs -n1 composer cs-fix ``` 1. `git diff --name-only --cached`:这部分命令用于获取你已经暂存(staged)的修改文件的列表。 2. `grep '\.php$'`:通过管道将文件列表传递给grep命令,这将只选择扩展名为 `.php` 的文件。 3. `xargs -n1 composer cs-fix`:xargs命令将每个文件名作为参数传递给 `composer cs-fix` 命令,从而格式化每个文件的代码。 ### 格式化某次提交的代码 ```shell git diff-tree --no-commit-id --name-only -r | grep '\.php$' | xargs -n1 composer cs-fix ``` 1. `git diff-tree --no-commit-id --name-only -r `:这部分命令获取了特定提交记录中的修改文件列表。 > **** 可以从 `git log` 获取 2. `grep '\.php$'`:通过管道将文件列表传递给 `grep` 命令,这将只选择扩展名为 `.php` 的文件。 3. `xargs -n1 composer cs-fix`:`xargs` 命令将每个文件名作为参数传递给 `composer cs-fix` 命令,从而格式化每个文件的代码。